Why Java Rocks
Some days ago I browsed a Kinisoftware slideshow and he said that “Java rules”. He couldn’t explain why Java rules at the end, so I decided to do it in his place.
But at the end I will explain why Java does not rule.
I hope that Kinisoftware can read this and criticate it with all his soul. Kini: This is for you!
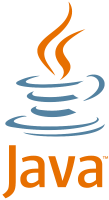
Java does rule
Static typing
The first thing you realize about Java is its static typing. This kind of typing has some advantages: it is more dificult to break anything and it can be used as documentation.
And Static Typing allows some things like:
Method overloading
Two functions with different parameters can be distinguised by their arguments, because of the type or the number of them. So, every call will be processed by the method that exactly matches with the calling arguments.
Using different parameters to select the method to be used avoids loads of unnecessary conditions and it is easier to follow the Single Responsability Principle (RSP): each method works only with one data type and other method with another type.
Polimorfism
We can overwrite a method in a child class, so it can behave in a different way than its parent.
Compiled
During the compilation stage, it performs some checks that are not necessary to be performed while the program is running, like argument validation. This should mean a faster and more reliable code.
Binary and packed
Compiled code is binary (bytecode), what makes easier to distribute the program. In addition, it can be packed in JARs or WARs, to make its distribution even easier.
These tools make the code organization easier too.
IDEs
You can find high quality IDEs. In fact, static typing allows the IDEs to be right with their proposals.
Checkers
There are a load of programs that allows different kind of analysis.
Libraries
Java’s standard library is wide, but there are a lot of libraries that allows you to make it even bigger, like Apache Commons.
Web oriented
There are lots of frameworks to write web programs: struts, spring, JSF,…
Documentation
Documentation uses to be quite good, and it can be embedded inside the IDE itself.
Speed
Opposite to what it has been said about Java, it is fast. Very fast. The main problem usually is the JVM load.
Version 1.4 was horrible, but using it to compare with other options is like comparing it working in a 386.
Sublanguages
It is very easy to add other interpreted languages to our Java programs. In fact, Java has an API to allow this.
Because of this, there are loads of interpreters: IronPython, Groovy, Clojure,… Standard Java already contains a JavaScript interpreter (Rinho).
Memory management
Java performs an efficient memory management, with cycle detections and every optimization you can imaginate.
Java does not rule
Static typing
Static typing makes more difficult to build generic things. You cannot make a generic method without creating a class for each data type, and a common interface for the whole thing.
In addition, only one public class is allowed in each file, what means you need at east 4 files (the main one, the interface and a couple of specializations).
Methods overload
Despite you can overload methods, it fails from time to time, because you can use an object that matches different prototypes. Example:
|
|
Operator overload
Java do not support operator overload. This is a pity, because it is easier to understand an operator than a method call:
|
|
Interpreter
-It has no interpreter shell- The interpreter shell available, beanshell, is not a standard Java component. It would be quite useful to make proof of concepts or little programs.
Initial complexity
The minimal program is very complex: it requires a public class, a static and public method, with a fixed structure and a fixed name, that manages an static array. This is one of the reasons because Java is criticated as a language to learn to write programs.
IDEs
It is very easy to fall in the IDEs trap and use its high capabilites for everything. So, we forget that we are importing too many classes or making a mess of architecture.
Distribution
Code distribution can be our worst enemy, because it is very easy to create cyclic dependencies that programs like Maven cannot solve.
Compilation
Compilation is a slow process and it does not apply code optimizations. This is something I do not understand, because I thought it was one of the better advantages.
It is true that there are other programs that allows you to optimize the bytecode, but I do not understand why the Java compiler is too slow to perform a “simple” code translation.
Memory
Despite Java performs a lot of checks, you cannot negate that Java programs require a lot of requirements.
Hosting
Java Hosting requires a complete virtual machine, what makes its price over 60€.
In pay per use systems, Java may be expensive.
Easy
Almost everything is XML based. XML is easy for the machine, but requires too much resources to be processed and is not so easy for human beings.
In addition, the only fact of opening a file is too complex. Last Java versions added the FileReader type, that makes this task easier, but there are a lot of APIs that still require an Stream, making the FileReader type not useful and forcing you to use the older way.
Another issue are dates: it is a pain to work with them.
And getters/setters: how long should we fight with them? I do not understand why I have to complicate a class when it can be easier.
Some examples of widely spread Java utilities that use XML:
Deprecated
There are a load of deprecated classes in the API, e.g. when we work with dates. Even more: there are a lot of modern machines that allows to run Java code, but they only allow old versions, like 1.4 or 1.5.
Incompetence
Despite there are huge professionals in Java world, it seems that everything is so easy that incompetence is encouraged. Maybe this is the mean reason why big corporations prefer Java to other languages.
Java allows a very appropiate use, applying patterns, reusing, etc. But big corporations prefer to have monkeys typing code and it seems that Java is the right thing to do that.
Please, if you use Java, don’t be a monkey.
Files
It is terrible to see how the amount of files is increased when we work with Java. Usually it is dificult to follow so many files without an IDE.
It is very easy to find developers trying to overload one class functionality only to try to avoid another new file. For sure: object orientation can only be understood when you apply the Single Responsability Principle.
The End
There are loads of reasons to use and to not use Java. I only tried to put them together here. I’m sure I’m forgetting a lot of them.
If you have any new, please, tell me. Do not worry if you are backbiting yourself: it is always good to know your tools scope.
And it is exactly why the next article will be “Why Python Rocks”.